Not every user wants to insert a password everytime to login to your app. A very common and handy feature is to use the phones biometric features like face unlock or fingerprint unlock. Titanium offers an open-source module named Ti.Identiy (https://github.com/tidev/titanium-identity) to do exactly that.
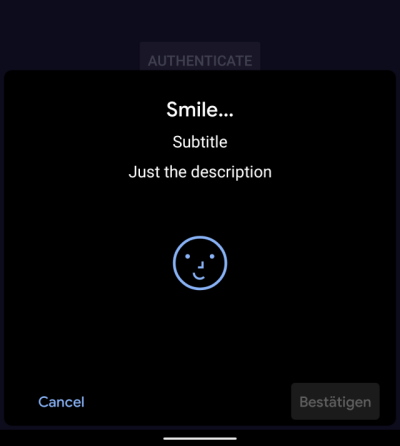
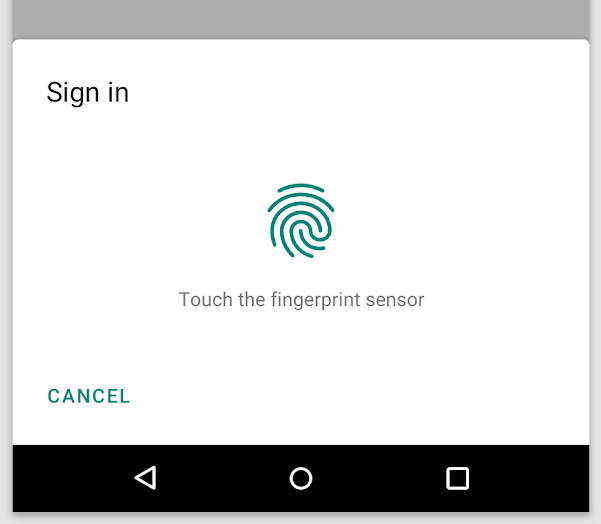
App setup
We create a new app with ti create
, follow that menu and then go into the folder and run alloy new
to make it an Alloy app.
After that we download the module from https://github.com/tidev/titanium-identity/releases and place the ZIP file in the root of your project. In your tiapp.xml
you’ll see a <modules></modules>
section and we add the name of the module in there like this:
<modules>
<module>ti.identity</module>
</modules>
and compile the app ti build -p android
. This will extract the module automatically and gets your gradle or Xcode running – future builds will be faster!
Note: in this tutorial I’ll use Android but you can use everything for iOS too and check it to -p ios
if you like.
UI
In order to trigger the unlock screen we simply create a Window with a Button. To do that we just use add this into app/views/index.xml
<Alloy>
<Window>
<Button title="unlock" onClick="doClick"/>
</Window>
</Alloy>
and this in your app/controllers/index.js
function doClick(e) {}
$.index.open();
When you run your app again you’ll see a simple button like this:
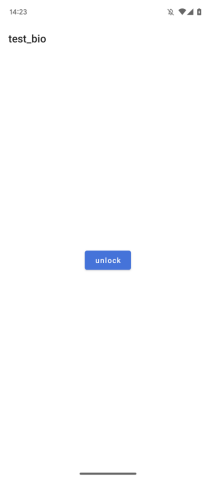
Logic
In the last step we just need to load the module and display the unlock screen. First is to require the module so you can use it in your controller:
const TiIdentity = require("ti.identity");
Then it is always good to check if the device actually has biometric features. If not we just ignore it and inform the user. Extend the doClick()
function:
function doClick(e) {
if (!TiIdentity.isSupported()) {
alert("Touch ID is not supported on this device!");
return;
}
}
Now we can call TiIdentity.authenticate()
with some parameters to display the unlock screen. The full controller looks like this now:
const TiIdentity = require("ti.identity");
function doClick(e) {
if (!TiIdentity.isSupported()) {
alert("Touch ID is not supported on this device!");
return;
}
TiIdentity.authenticate({
reason: 'Unlock with biometric feature',
reasonSubtitle: "Please unlock",
reasonText: "To access the app please unlock",
cancelTitle: "Cancel",
callback: function(e) {
if (!e.success) {
alert('Message: ' + e.error);
} else {
alert('YAY! success');
}
}
});
}
$.index.open();
The full list of methods and properties is available in the official docs at https://titaniumsdk.com/api/modules/identity.html. Check that for more examples and how to use it to access the Keychain.